import seaborn as sns
import matplotlib.pyplot as plt
sns.set_theme(style="whitegrid")
iris = sns.load_dataset("iris")
iris

데이터셋 설명
- 아이리스(Iris) 데이터셋은 세 가지 종류의 붓꽃(setosa, versicolor, virginica)에 대한 네 가지 측정값(꽃받침 길이/너비 및 꽃잎 길이/너비)을 포함하고 있습니다:
- species: 붓꽃의 종류 (Setosa, Versicolor, Virginica).
- sepal_length: 꽃받침 길이.
- sepal_width: 꽃받침 너비.
- petal_length: 꽃잎 길이.
- petal_width: 꽃잎 너비.
- 시각화에서는 이 네 가지 측정값을 하나의 축(measurement)으로 통합하고, 각 측정값에 대한 붓꽃 종류별 분포를 보여줍니다.
seaborn
# "Melt" the dataset to "long-form" or "tidy" representation
iris = iris.melt(id_vars="species", var_name="measurement")
iris
# Initialize the figure
f, ax = plt.subplots()
sns.despine(bottom=True, left=True)
# Show each observation with a scatterplot
sns.stripplot(
data=iris,
x="value",
y="measurement",
hue="species",
dodge=True,
alpha=.25,
zorder=1,
legend=False,
)
# Show the conditional means, aligning each pointplot in the
# center of the strips by adjusting the width allotted to each
# category (.8 by default) by the number of hue levels
sns.pointplot(
data=iris,
x="value",
y="measurement",
hue="species",
dodge=.8 - .8 / 3,
palette="dark",
errorbar=None,
markers="d",
markersize=4,
linestyle="none",
)
# Improve the legend
sns.move_legend(
ax, loc="lower right",
ncol=3,
frameon=True,
columnspacing=1,
handletextpad=0,
)
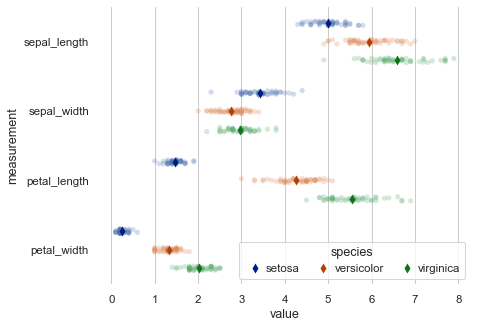
주요 구성 요소
- 데이터 전처리:
- iris.melt(id_vars="species", var_name="measurement"): 아이리스 데이터셋은 원래 sepal_length, sepal_width, petal_length, petal_width라는 네 가지 측정값을 각각 열로 가지고 있습니다. 이를 long-form 형식으로 변환하여 measurement라는 열에 각 측정값의 이름을 넣고, value 열에 그 값을 넣습니다. 이렇게 하면 시각화할 때 더 유연하게 사용할 수 있습니다.
- 스트립 플롯(Stripplot):
- sns.stripplot(): 각 데이터 포인트를 점으로 표현하는 플롯입니다. 여기서는 각 측정값(measurement)에 대해 해당 값(value)을 x축에 배치하고, 종(species)에 따라 색상을 구분합니다.
- dodge=True: 종별로 점들을 서로 분리하여 시각적으로 구분하기 쉽게 만듭니다.
- alpha=.25: 점들의 투명도를 0.25로 설정하여 겹치는 부분을 덜 강조합니다.
- zorder=1: 스트립 플롯이 포인트 플롯보다 뒤에 그려지도록 설정합니다.
- sns.stripplot(): 각 데이터 포인트를 점으로 표현하는 플롯입니다. 여기서는 각 측정값(measurement)에 대해 해당 값(value)을 x축에 배치하고, 종(species)에 따라 색상을 구분합니다.
- 포인트 플롯(Pointplot):
- sns.pointplot(): 각 종의 평균 값을 점으로 나타내는 플롯입니다. 스트립 플롯 위에 각 종의 평균값이 중심에 오도록 정렬됩니다.
- dodge=.8 - .8 / 3: 종별로 점들을 분리하여 표시합니다.
- markers="d": 다이아몬드 모양 마커를 사용합니다.
- markersize=4: 마커 크기를 4로 설정합니다.
- linestyle="none": 선 없이 점만 표시합니다.
- sns.pointplot(): 각 종의 평균 값을 점으로 나타내는 플롯입니다. 스트립 플롯 위에 각 종의 평균값이 중심에 오도록 정렬됩니다.
- 범례(Legend) 조정:
- sns.move_legend(): 범례를 그래프 우측 하단에 배치하고, 한 줄에 3개의 항목이 들어가도록 설정합니다.
- frameon=True: 범례에 테두리를 추가합니다.
적용 사례
- 생물학 연구:
- 생물학자들은 다양한 붓꽃의 측정값 분포를 비교하여 종 간 차이를 분석할 수 있습니다. 예를 들어 특정 붓꽃 종류가 다른 종보다 꽃잎이나 꽃받침이 더 긴지 확인할 수 있습니다.
- 교육용 데이터 시각화:
- 아이리스 데이터셋은 머신러닝이나 통계학 교육에서 자주 사용되며, 이와 같은 시각화를 통해 데이터를 직관적으로 이해할 수 있도록 도울 수 있습니다.
- 데이터 분석 및 보고서 작성:
- 이와 같은 시각화는 보고서나 프레젠테이션에서 데이터를 요약하고 주요 패턴을 강조하는 데 유용합니다.
'[업무 지식] > Seaborn' 카테고리의 다른 글
[jointplot] Joint kernel density estimate (0) | 2024.11.13 |
---|---|
[Jointgrid] Joint and marginal histograms (0) | 2024.11.13 |
[boxplot] Horizontal boxplot with observations (0) | 2024.11.09 |
[histplot] Stacked histogram on a log scale (0) | 2024.11.09 |
[jointplot] Hexbin plot with marginal distributions (1) | 2024.11.09 |